Title: Implementing Bubble Sort in C#: A Step-by-Step Guide
Introduction:
Bubble sort is one of the simplest sorting algorithms used to arrange elements in an array or list in a specific order. It works by repeatedly iterating through the data, comparing adjacent elements and swapping them if they are in the wrong order. In this post, we’ll explore how to implement bubble sort in C# and understand its time and space complexity.
The Algorithm:
Here is the C# implementation of the Bubble Sort algorithm:
using System;
public class BubbleSort
{
public static void Sort(int[] array)
{
int n = array.Length;
bool swapped;
for (int i = 0; i < n - 1; i++)
{
swapped = false;
for (int j = 0; j < n - i - 1; j++)
{
if (array[j] > array[j + 1])
{
// Swap elements
int temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
swapped = true;
}
}
// If no swaps were made, the array is already sorted
if (!swapped) break;
}
}
}
Explanation:
The Sort
method takes an integer array as input and sorts it using the Bubble Sort algorithm. Here’s a step-by-step explanation of how it works:
- The outer loop iterates
n-1
times, wheren
is the length of the input array. - In each iteration of the outer loop, the inner loop iterates from the first element to the second last element (i.e.,
n-i-1
elements). - In the inner loop, adjacent elements are compared. If an element is greater than its next element, they are swapped using a temporary variable.
- A boolean flag
swapped
is set totrue
if any swaps were made in the inner loop. - If no swaps were made in the entire iteration of the outer loop, it means the array is already sorted, and we can exit the algorithm.
Time Complexity:
The time complexity of Bubble Sort is O(n^2) in the worst case, where n
is the length of the input array. This is because the algorithm has to iterate through the entire array multiple times
to ensure all elements are in the correct order.
Space Complexity:
The space complexity of Bubble Sort is O(1), which means it requires a constant amount of additional memory to perform the sorting operation.
Example Usage:
Here’s an example usage of the BubbleSort
class:
int[] array = { 5, 2, 8, 3, 1, 6, 4 };
BubbleSort.Sort(array);
Console.WriteLine("Sorted array:");
foreach (int element in array)
{
Console.Write(element + " ");
}
Conclusion:
In this post, we’ve implemented the Bubble Sort algorithm in C# and explored its time and space complexity. While it’s not the most efficient sorting algorithm, Bubble Sort is simple to understand and implement, making it a great introduction to sorting algorithms for beginners.
I hope you found this tutorial helpful! Let me know if you have any questions or need further clarification on any of the concepts discussed in this post. Happy coding!
使用微信扫描二维码完成支付
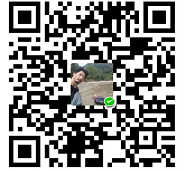