Using Moq for Mocking in C#
Mocking is an essential technique in software development, especially in unit testing, where dependencies need to be replaced with test doubles to isolate the unit under test. Moq is a popular mocking library for C# that provides a fluent interface for creating mock objects. In this guide, we’ll explore the basics of using Moq to create mock objects.
Installation
First, ensure you have the Moq package installed in your project. You can install it via NuGet Package Manager Console:
Install-Package Moq
Or using the .NET Core CLI:
dotnet add package Moq
Basic Usage
Let’s say we have an interface IFoo and a class Bar that depends on IFoo.
public interface IFoo
{
string GetMessage();
}
public class Bar
{
private readonly IFoo _foo;
public Bar(IFoo foo)
{
_foo = foo;
}
public string Greet()
{
return "Hello, " + _foo.GetMessage();
}
}
Now, we want to test the Bar class without depending on the actual implementation of IFoo. We can use Moq to create a mock object for IFoo.
using Moq;
// Create a mock object for IFoo
var fooMock = new Mock<IFoo>();
// Setup the behavior of the mock
fooMock.Setup(foo => foo.GetMessage()).Returns("World");
// Inject the mock object into Bar
var bar = new Bar(fooMock.Object);
// Perform the test
string result = bar.Greet();
// Verify the interaction
fooMock.Verify(foo => foo.GetMessage(), Times.Once);
In the above example:
-
We create a mock object for IFoo using Mock().
-
We set up the behavior of the mock using Setup(), specifying the method to mock and its return value.
-
We inject the mock object into an instance of Bar.
-
We perform the test by calling the Greet() method.
-
Finally, we verify that the GetMessage() method was called exactly once on the mock object.
Conclusion
Moq provides a convenient way to create mock objects for testing in C#. By using Moq, you can easily isolate the units under test and focus on testing their behavior without worrying about the actual implementations of their dependencies.
使用微信扫描二维码完成支付
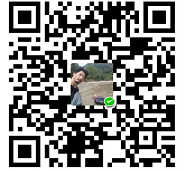