Step-by-Step Guide to Calling Google Indexing API to Publish URL Notifications with C#
Google Indexing API is a powerful tool that allows website owners to notify Google about changes to their website’s content. This article will guide you through the process of using C# language and Google API client library to call Google Indexing API for publishing URL notifications.
Step One: Preparation
First, we need to prepare the following:
-
Install Google API Client Library: Install the
Google.Apis
andNewtonsoft.Json
packages using NuGet Package Manager. -
Obtain Google OAuth 2.0 Client Credentials: Create OAuth 2.0 client credentials in Google Cloud Console, download the JSON file, and save it locally.
Step Two: Write Code
Next, we’ll write C# code to call Google Indexing API.
using Google.Apis.Auth.OAuth2;
using Google.Apis.Indexing.v3;
using Google.Apis.Indexing.v3.Data;
using Google.Apis.Services;
using Newtonsoft.Json;
using System.Threading;
// Load Google OAuth2 client credentials
GoogleClientSecrets secrets = GoogleClientSecrets.FromFile("/path/to/client_secret.json");
// Get Indexing API credentials
UserCredential credential = GoogleWebAuthorizationBroker.AuthorizeAsync(
secrets.Secrets,
new[] { "https://www.googleapis.com/auth/indexing" },
"user",
CancellationToken.None).Result;
// Initialize Indexing service
var service = new IndexingService(new BaseClientService.Initializer
{
HttpClientInitializer = credential
});
// Create URL notification object
UrlNotification notification = new UrlNotification { Url = "http://example.com/", Type = "URL_UPDATED" };
// Publish URL notification
UrlNotificationsResource.PublishRequest request = service.UrlNotifications.Publish(notification);
var response = request.Execute();
// Print API call response
Console.WriteLine(JsonConvert.SerializeObject(response));
Step Three: Code Analysis
The code above follows these steps:
-
Load Google OAuth2 client credentials, including client ID and client secret.
-
Obtain credentials for Indexing API using OAuth 2.0 client credentials.
-
Initialize Indexing service with obtained credentials for authentication.
-
Create a URL notification object, specifying the URL to be updated.
-
Publish the URL notification using the Indexing service.
-
Get the response of the API call, serialize it to JSON format, and output it to the console.
Conclusion
Through this article, we have provided a detailed guide on how to use C# language and Google API client library to call Google Indexing API for publishing URL notifications. By correctly configuring OAuth 2.0 client credentials and making the right API calls, you can effectively notify Google about updates to your website’s content, helping it discover and index your website content faster.
使用微信扫描二维码完成支付
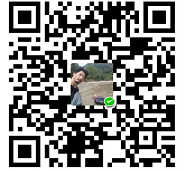